Introduction
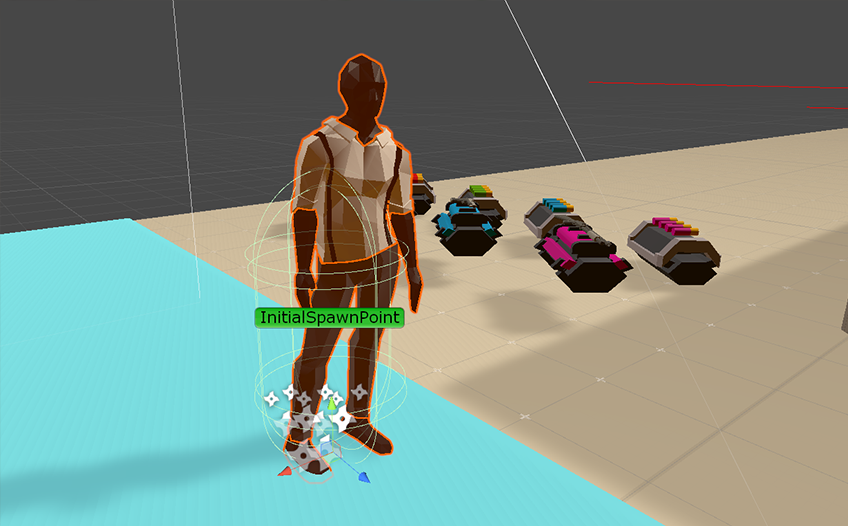
“Agents” in the TopDown Engine is a term used to describe any kind of characters, whether they’re playable characters, or enemies, NPCs, etc. There are a few core classes that make these agents work, and that you’ll need to get familiar with if you want to expand and create more character abilities for example.
In the meantime, this page aims at presenting the basic concepts and allowing you to quickly create your own character (player controlled or AI based).
Creating a basic 2D playable character
- in Unity 6000.0.23f1 (or higher), create a new project and import TopDown Engine v4.2 via the Package Manager
- open Minimal2D demo scene
- create a new, empty game object, position it at 40,-20,0, call it Test
- create a new sprite object, set its Sprite to Adventurer_0, sorting layer to Characters, nest it under Test, at 0,0,0
- on Test, add a Character component
- press AutoBuild Player Character 2D
- drag the NewSprite into the CharacterModel slot
- under CharacterOrientation2D, set facing mode to MovementDirection, check Model Should Flip
- open the Koala prefab, copy the footsteps node
- paste in your scene
- nest it under Test, at 0,0,0
- press play
Creating a basic 3D playable character
- in Unity 6000.0.23f1 (or higher), create a new project and import TopDown Engine v4.2 via the Package Manager
- open MinimalScene3D demo scene
- create a new, empty game object, position it at 0,0,0, call it Test
- create a new cube, drag it under Test, position it at 0,0,0, name it Model, remove its BoxCollider
- on Test, add a Character component
- press the AutoBuild Player Character 3D button at the bottom of its inspector
- on the CharacterController component, set Height to 1
- on the TopDownController3D component, set UpdateMode to Update
- on the Character component, drag the Model into the CharacterModel slot
- under CharacterOrientation3D, set Rotation Mode to MovementDirection, drag the Model into the Movement Rotating Model slot
- drag that Test object in your Project panel to make it a prefab, remove it from the scene
- select the LevelManager, drag that new prefab into the first array entry of PlayerPrefabs
- press play, enjoy your new friend
Extra steps : adding an Animator
- on the Model object inside your character, add an Animator component
- in your Project panel, create a new AnimatorController, name it TestAnimatorController, drag it into the Animator’s Controller slot
- on the same object, add a CharacterAnimationParametersInitializer component, press its AddAnimationParameters button (the component disappears, that’s normal)
- at the top level of your character, drag the Model into the Character component’s CharacterAnimator slot
- save your prefab, press Play
- in the Hierarchy panel, select the Model object, double click on its Controller in its Animator component, this will open the Animator panel, there you can see that its various animation parameters update. You can use all of these to setup your transitions between animations.
Creating a basic 2.5D playable character
If you want to create a character that evolves in a 3D world, but appears as a sprite (think Paper Mario), you can do it like so :
- in Unity 6000.0.23f1 (or higher), create a new project and import TopDown Engine v4.2 via the Package Manager
- open MinimalScene3D demo scene
- create a new, empty game object, position it at 0,0,0, call it Test
- create an empty child, name it Model
- create a new sprite renderer, set its sprite to Koala_Idle_0, drag it under Model, position it at 0,0,0, name it MySprite
- on Test, add a Character comp
- press AutoBuild Player Character 3D
- on Test’s Character component, drag the Model node into the CharacterModel slot
- on Test, remove the CharacterOrientation3D component, add a CharacterOrientation2D, set FacingMode:MovementDirection, ModelShouldFlip:true
- on MySprite, add a MMBillboard component, NestObject:false, OffsetDirection:0,0,1
- drag the Test object in your Project panel to make it a prefab, remove it from the scene
- select the LevelManager, drag that new prefab into the first array entry of PlayerPrefabs
- press play, enjoy your new 2.5D friend
Creating a basic 3D enemy
- in Unity 6000.0.23f1 (or higher), create a new project and import TopDown Engine v4.2 via the Package Manager
- open Loft3D demo scene
- create an empty game object, set it at -9.5,1.5,0
- create a cube, set its scale to 2,2,2, set it under the empty
- rename the empty to “Test”
- on Test, add a Character component
- press the Autobuild AI Character 3D button
- in the Character inspector, set the CharacterModel to the Cube
- press play, grab the shotgun next to you, enjoy
Base concepts
The TopDown Engine is designed to work for both 2D and 3D projects. The components you’ll use to create characters for one setup or the other will be mostly the same, but some will be specific to 2D or 3D. When you encounter a component or an ability, if it doesn’t have 2D or 3D in its name, it’s safe to assume it’ll work for both. Otherwise, you’ll want to use components with 2D in their name for 2D only, and components with 3D in their name for 3D only.
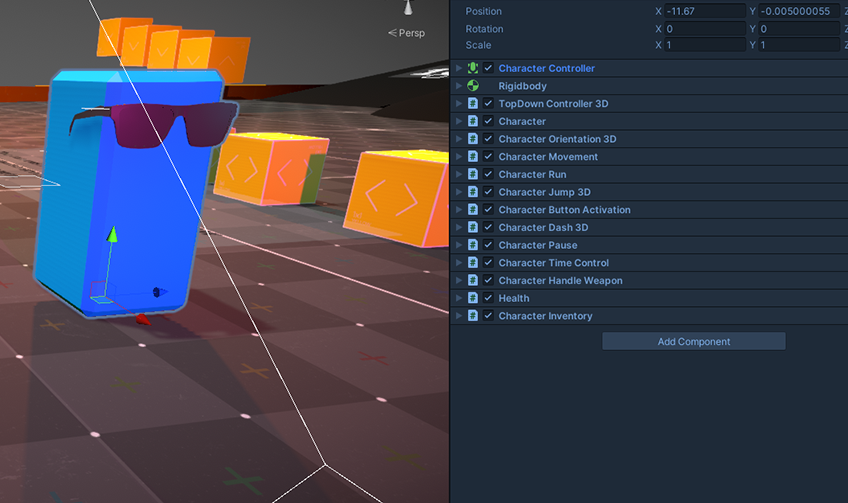
This page goes into more details about the mandatory components of a Character, but here’s a brief rundown. An agent in the TopDown Engine usually has these components :
- A Collider : BoxCollider2D or CircleCollider2D in 2D, CharacterController in 3D, this collider’s size is used to determine collisions and where in the world the agent is.
- RigidBody2D or 3D : only used to provide basic interactions with standard physics (completely optional)
- TopDownController : responsible for collision detection, basic movement (move left / right), gravity, the TopDownController is your character’s motor class. It comes in both 2D and 3D versions, but both share the same methods and logic. Note that the 3D version requires a CharacterController component.
- Character : This is the central class that will link all the others. It doesn’t do much in itself, but really acts as a central point. That’s where you define if the player is an AI or player-controlled, where its model and animator are, stuff like that. It’s also the class that will control all character abilities at runtime.
- Health : Not mandatory, but in most games your agents will be able to die. The Health component handles damage, health gain/loss, and eventually death.
- Character Abilities : So far all the previous components offer lots of possibilities, but don’t really “do” anything visible. That’s what Character Abilities are for. The asset comes packed with more than 15 abilities, from simple ones like CharacterMovement to more complex ones like weapon handling. They’re all optional, and you can pick whichever you want. You can of course also easily create your own abilities to build your very own game.
Hierarchy
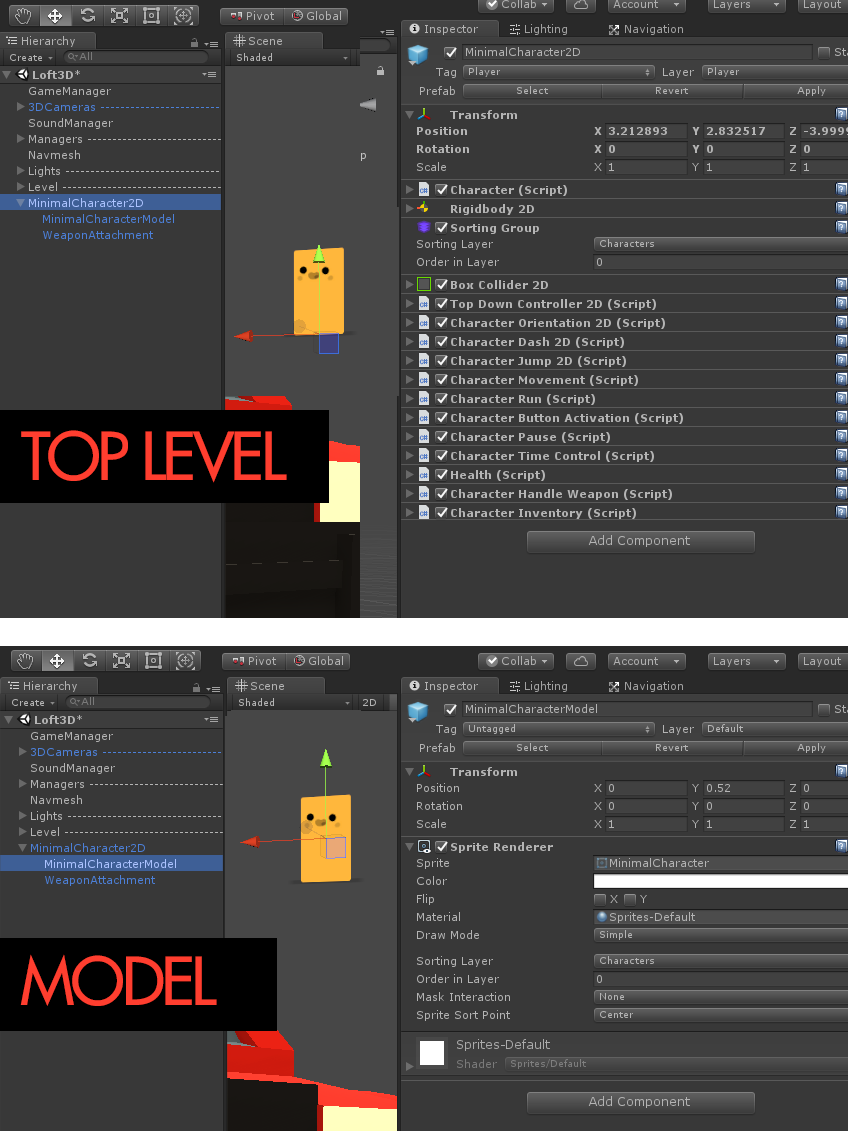
However you create your character, one thing that is really important is to understand how to structure it. You’ll want to separate the logic (the RigidBody, TopDownController, Character abilities, etc) from the visuals (your model / sprite / Spine setup, etc). You can have many more layers (maybe your animator sits on its own layer, etc), but the recommended setup is the one on the image above : a top level with all the logic, and the model nested inside it.
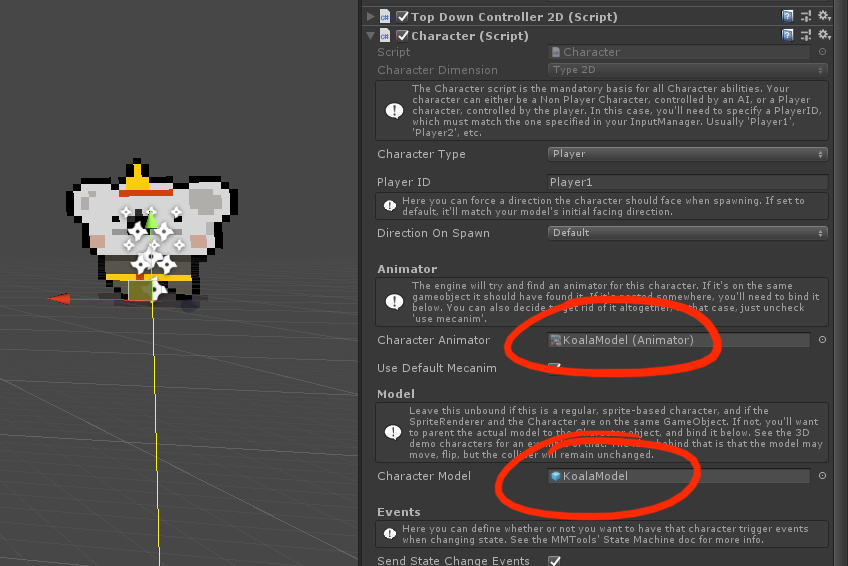
Make sure you link the Model to the Character class, same for the animator, from the inspector. Some other classes (Orientation abilities, Health) may also require you to tell them, via their inspector, where the Model or Animator is.
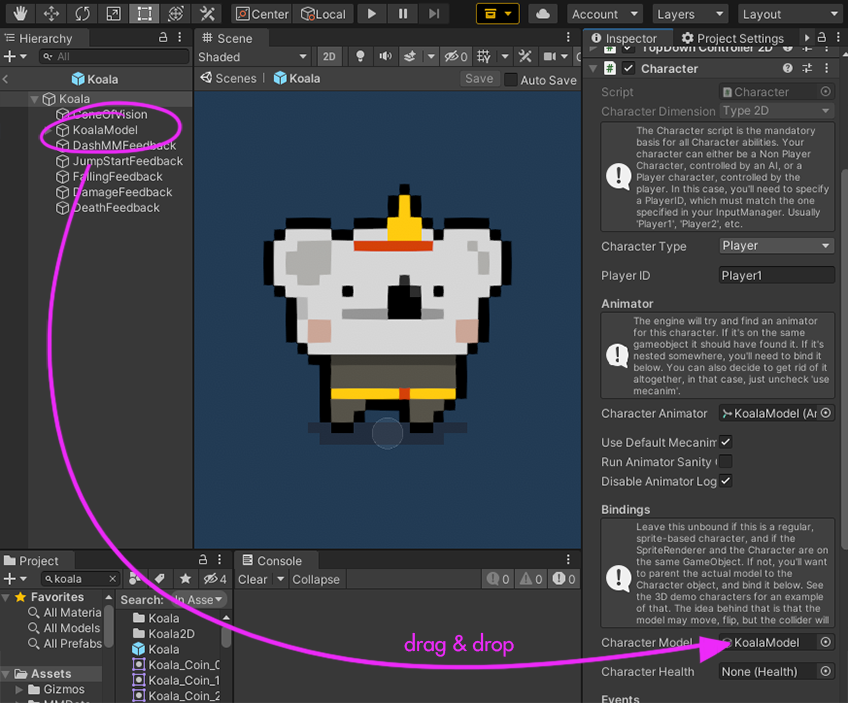
How do I create an Agent ?
There are many ways you can create a playable or AI character in the TopDown Engine. Here we’ll cover the 3 recommended ones. Note that if you prefer doing differently, as long as it works for you, it’s all fine.
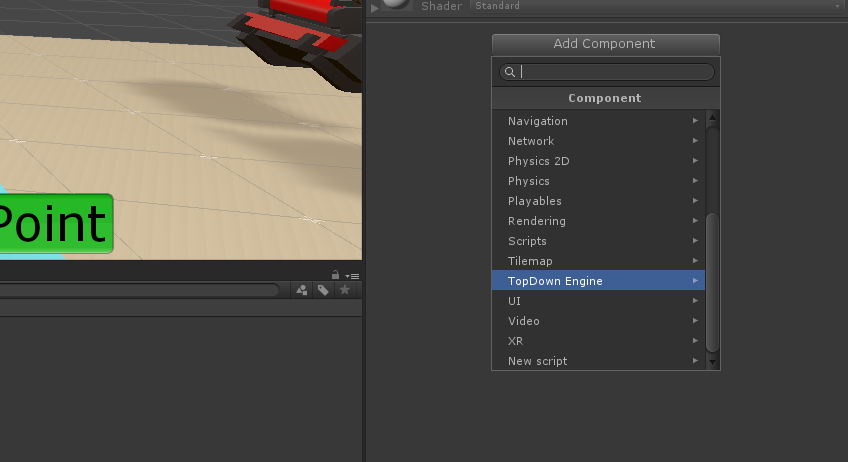
Automatic Creation
The “Autobuild Character” feature allows you to create a new character in a few seconds. Note that after that initial setup you’ll still have to setup animations and all that.
Here’s how to proceed :
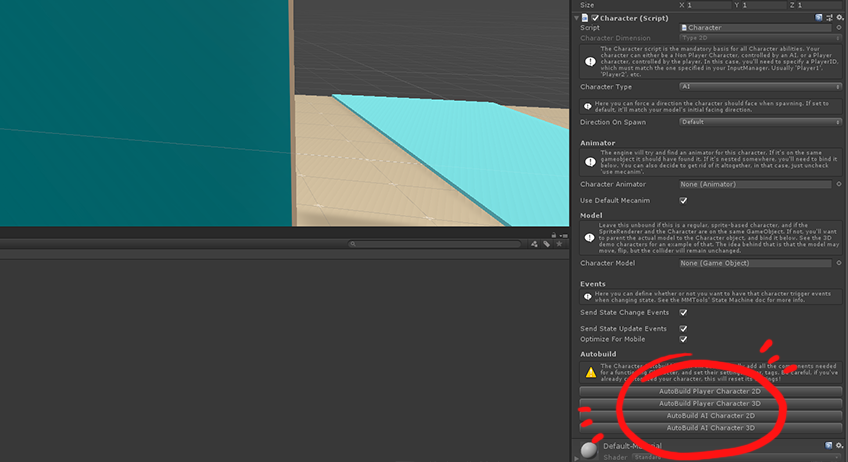
For 3D :
- open the MinimalScene3D demo scene (or any scene that meets the minimal requirements
- create an empty game object, name it “Test”, position at 0,0.5,0
- under it, add a Cube, center it, scale it to 1,2,1, remove its BoxCollider
- on Test, add a Character comp, drag the Cube in its CharacterModel slot
- on the Character inspector, press Autobuild Player Character 3D
- press play
For 2D :
- open the Minimal2D demo scene (or any scene that meets the minimal requirements
- create an empty game object, name it “Test”, position it in the scene
- under it, add a game object, center it, name it “Model”, add a sprite renderer to it and set its sprite
- on Test, add a Character comp, drag the Model object you just created in its CharacterModel slot
- on the Character inspector, press Autobuild Player Character 2D
- press play
Of course it’d be the same thing for AIs, except you’d pick Autobuild AI Character 2D/3D. If you went for an AI character, it’s ready to get a Brain and some Actions and Decisions. You can now fine tune the various settings, remove the abilities you’re not interested in for this character, add animations, etc. Or you can leave it like that and start prototyping the rest of your game and levels.
Copy
Another fast way to create an agent is to find one you like in the demos, and create yours from that. The process for that is quite simple :
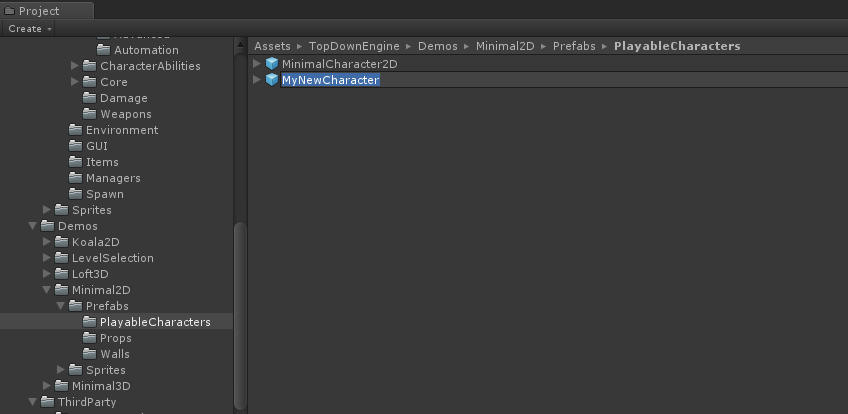
- Find an agent you like in one of the demos.
- Locate its prefab (select the Agent in Scene View, and in its inspector, at the very top right under the prefab’s name and tag there’s a Select button)
- Duplicate the prefab (cmd + D)
- Rename it to whatever you want your Character to be called
- Make the changes you want. Maybe you’ll just want to replace some settings, maybe you’ll want to change the sprite and animations. It’s up to you from there.
Components approach
You can also create a Character from scratch. It’s longer but why not?
- Start with an empty gameobject. Ideally you’ll want to separate the Character part from the visual part. The best possible hierarchy has the TopDownController/Collider/Character/Abilities on the top level, and then nests the visual parts (sprite, model, etc).
- At the top of the inspector, set the tag to Player if it’s a player character, or to anything you prefer if it’s not. Same thing for the layer.
- On your top level object, add a Collider (either 2D or 3D, we recommend a Capsule for 3D characters, a box for 2D ones). Adjust its size to match your sprite/model dimensions. Then add a RigidBody2D or RigidBody component.
- Add a TopDownController (2D or 3D) component. Set the various settings (see the class documentation if you need help with that), and make sure to set the various collision masks (platforms, one ways, etc). Add a CharacterController component too if you’re going for 3D.
- Add a Character component. Check the various settings to make sure they’re ok with you.
- Add the Character Abilities you want (it’s best to use the AddComponent button at the bottom of the inspector for that, and navigate there)
- Optionnally, add a Health component, a HealthBar component, etc.